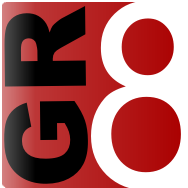
Craig Burke
Groovy/Grails Developer at Carnegie Mellon
Creator of Various AngularJS Related Build Tools
Creator of AngularJs/Grails Lazybones Template
www.craigburke.com
@craigburke1
Let’s Build An Angular App
Gradle (and various plugins)
Asset Pipeline (and various modules)
John Papa’s Angular Style Guide
That’s About It
plugins {
id 'com.bertramlabs.plugins.asset-pipeline' version '2.4.2'
}
task build(dependsOn: ['assetClean', 'assetCompile'])
assetCompile.shouldRunAfter 'assetClean'
Client-Side Dependencies
Download and Manage Dependencies Ourselves
Use Npm or Bower with Grunt or Gulp
Use Gradle with the Bower Installer Plugin
Getting Started
plugins {
id 'com.moowork.node' version '0.10'
id 'com.craigburke.bower-installer' version '1.3.3'
}
bowerInstall installs dependencies
bowerClean removes dependencies
bowerRefresh removes and reinstalls dependencies
bower {
dependencies = [
bootstrap: '3.3.x'
]
sources = [
bootstrap: ['dist/css/bootstrap.min.css', 'dist/fonts/**']
]
excludes = ['jquery']
}
Add dependency to dependencies list
bowerRefresh -PbowerDebug=true
Limit files with the sources properties
Limit transitive dependencies with excludes
bowerRefresh
JavaScript Minification
function ListController($log) {
$log.error('HELP!');
}
myApp.controller('ListController', ListController);
function a(b) {
b.error('HELP!');
}
c.controller('ListController', a);
function ListController($log) {
$log.error('HELP!');
}
ListController.$inject = ['$log'];
myApp.controller('ListController', ListController);
function ListController($log) {
$log.error('HELP!');
}
myApp.controller('ListController', ['$log', ListController]);
Manually use $inject or inline annotations
Add special @ngInject comments to our functions
Use the Angular Annotate Asset Pipeline plugin
apply plugin: 'asset-pipeline'
assets {
minifyOptions = [
angularPass: true
]
}
// @ngInject
function ListController($log) {
$log.error('HELP!');
}
myApp.controller('ListController', ListController);
buildscript {
dependencies {
classpath 'com.craigburke.angular:angular-annotate-asset-pipeline:2.1.2'
}
}
Global Scope
Wrap Angular components in an Immediately Invoked Function Expression (IIFE)
(function() {
angular.module('myApp', []);
})();
Manually add a closure to every file
Use CoffeeScript
Use Js Closure Wrap Asset Pipeline Plugin
buildscript {
dependencies {
classpath 'com.craigburke:js-closure-wrap-asset-pipelin:1.1.0'
}
}
//= wrapped
angular.module('myApp', []);
Managing Templates
<div ng-include=" 'my-template.html' "></div>
If my-template.html isn’t in Angular’s template cache then an HTTP GET request is done for my-template.html
<script type="text/ng-template" id="my-template.html">
<h1>My template</h1>
</script>
myApp.run(function($templateCache) {
$templateCache.put('my-template.html', '<h1>My template</h1>');
});
Just use plain html template files
Add to $templateCache via a script tag or run block
Use the Angular Templates Asset Pipeline plugin
buildscript {
dependencies {
classpath 'com.craigburke.angular:angular-template-asset-pipeline:2.2.1'
}
}
<p>Hello</p>
angular.module('myApp.users').run(function($templateCache) {
$templateCache.put('/my-app/users/list.html', '<p>Hello</p>');
});
assets {
configOptions = [
angular : [ moduleNameBase: 'myApp' ]
]
}
<p>Hello</p>
angular.module('myApp.users').run(function($templateCache) {
$templateCache.put('/users/list.html', '<p>Hello</p>');
});
Running JavaScript Tests
Don’t write tests
Run test from Java using Nashorn or Rhino
Use the Jasmine Gradle Plugin
Getting Started
plugins {
id 'com.moowork.node' version '0.10'
id 'com.craigburke.jasmine' version '1.0.1'
}
jasmineRun Runs jasmine tests
jasmineWatch Runs jasmine tests in watch mode
jasmineClean Removes generated karma config file
jasmine {
files = [
'bower/angular/angular.js',
'bower/**/*.js',
'**/*.module.js',
'**/!(*.spec).js',
'**/*.spec.js'
]
}
Spring Boot, Grails and Ratpack
buildscript {
dependencies {
// Asset pipeline modules set as build dependencies
classpath 'com.craigburke.angular:angular-annotate-asset-pipeline:2.1.2'
classpath 'com.craigburke:js-closure-wrap-asset-pipeline:1.1.0'
classpath 'com.craigburke.angular:angular-template-asset-pipeline:2.2.1'
}
}
dependencies {
compile 'com.bertramlabs.plugins:asset-pipeline-spring-boot:2.4.2'
// Asset pipeline modules set as runtime dependencies
runtime 'com.craigburke.angular:angular-annotate-asset-pipeline:2.1.2'
runtime 'com.craigburke:js-closure-wrap-asset-pipeline:1.1.0'
runtime 'com.craigburke.angular:angular-template-asset-pipeline:2.2.1'
}
package angular
import org.springframework.boot.SpringApplication
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.context.annotation.ComponentScan
@SpringBootApplication
@ComponentScan('asset.pipeline.springboot')
class Application {
static void main(String[] args) {
SpringApplication.run Application, args
}
}
Good News! Everything works out of the box!
Any configuration settings should be in both the build.gradle and application.yml |
Asset Pipeline works well there too:
Books
AngularJS: Up and Running
ng-book
Pro AngularJs
Learning Ratpack (Dan Woods) - If he ever finishes it
Presentations
Asset-Pipeline by THE David Estes (later today)